Description
While not strictly necessary, utilizing a dependency injection framework can drastically simplify the management of dependencies between Android architectural components. This supports a clean decoupling of architectural components and avoids unnecessary boilerplate for connecting them. Doing so not only improves the maintainability of the app, but also improves its testability by providing the possibility to inject mock implementations. The Dagger framework is commonly recommended to inject dependencies and solve problems afflicting reflection-based solutions.
Introduction
Dependency injection is a technique whereby one object supplies dependencies of another object. So let’s say I’m a chef in a kitchen. And I need to make meals for the customers. But if I don’t have the tools to create my dishes, nothing happens. I crash. So we need someone to supply the tools, which is Dagger.
Dagger tells where all the dependencies are, and tells the objects not to worry. Because Dagger will provide the dependencies. To perform this dependency injection, dagger uses the following annotation:
- @Module and @Provides: These classes provide the dependencies.
- @Inject: The field which will be injected, this can be a field, a constructor or a method.
- @Component: Which uses the selected modules, and performs the dependency injection.
Example
We created a simple MVP login Application. The event diagram of the MVP login:
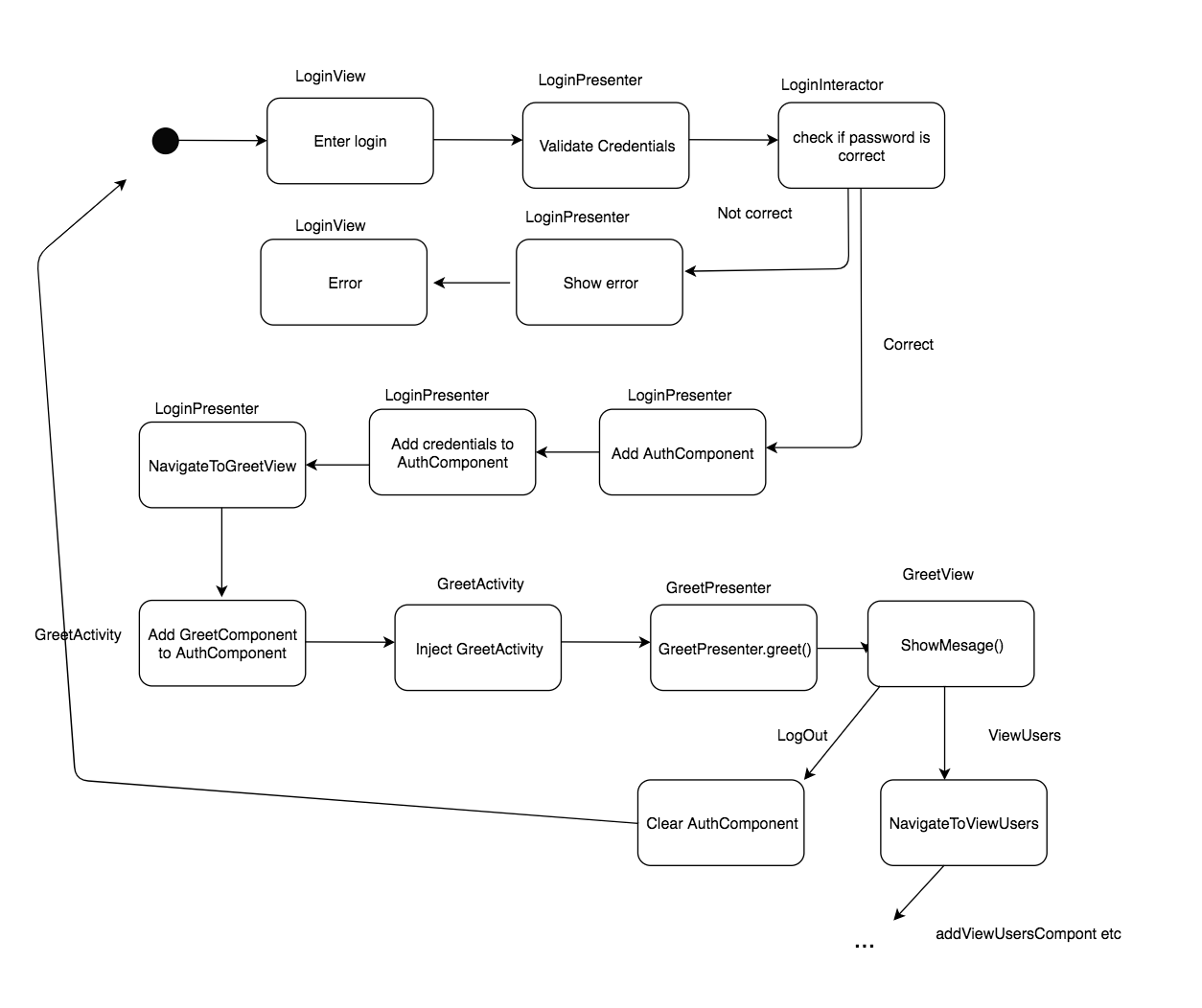
The lifecycle of our components:
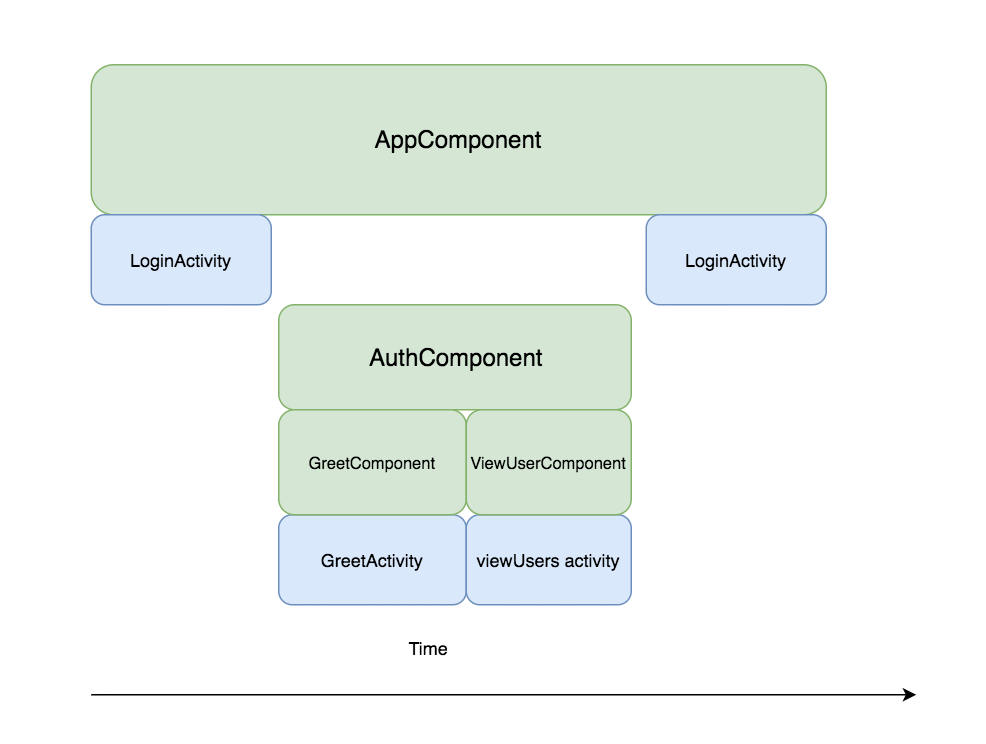
As you can see the AuthComponent gets added to the AppComponent after the validation of the credentials.
We will now focus on the AuthComponent. The AuthComponent uses the AuthModule. And allows two components to be added to this component:
- The GreetComponent
- The ViewUserComponent
The AuthModule provides whatever the AuthComponent needs. In this case the AuthComponent only needs to provide the credentials. So a provideCredentials method is added.
When the validation of the login has succeeded, the AuthComponent gets added to the AppComponent. Also the credentials from the AuthComponent are being set with the given username.
After this the GreetComponent gets added to the AuthComponent in the GreetActivity with the setupComponent method. And the GreetActivity is injected into the GreetComponent. Now everything the GreetActivity needs is taken care of in the greetModule.
Now the only job of the GreetPresenter is to greet the user with it’s username, by calling the mGreetView.showMessage(mCredentials.getPersonalizedGreeting()) method. We do not have to check if the username is set, because Dagger has provided the credentials.
Without Dagger, each component has to specify all its dependencies. And multiple null-checks have to be made before using that dependency..
Check out the Github page to view the complete repository.